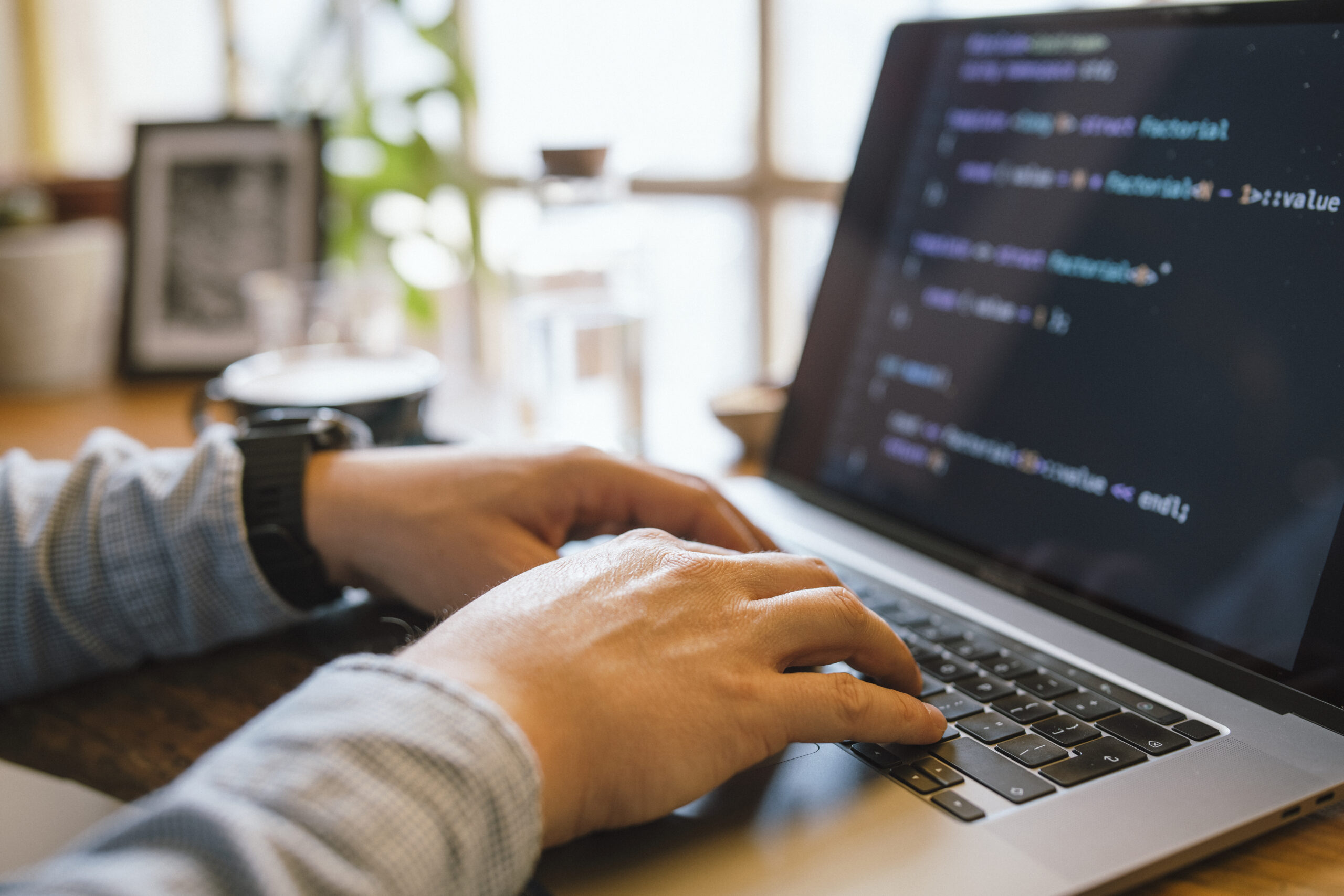
Debugging is Just about the most necessary — however usually forgotten — competencies in a developer’s toolkit. It isn't nearly repairing broken code; it’s about comprehension how and why points go Completely wrong, and Discovering to think methodically to solve problems efficiently. Whether or not you're a newbie or perhaps a seasoned developer, sharpening your debugging abilities can conserve hours of frustration and significantly enhance your productivity. Here are several procedures that will help builders stage up their debugging recreation by me, Gustavo Woltmann.
Master Your Tools
One of the fastest approaches developers can elevate their debugging abilities is by mastering the tools they use everyday. When producing code is a single Portion of development, recognizing tips on how to communicate with it successfully during execution is Similarly crucial. Modern enhancement environments appear equipped with impressive debugging capabilities — but many builders only scratch the surface area of what these applications can do.
Take, one example is, an Integrated Enhancement Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These resources assist you to set breakpoints, inspect the worth of variables at runtime, phase through code line by line, and in many cases modify code on the fly. When made use of accurately, they let you notice precisely how your code behaves through execution, which can be a must have for tracking down elusive bugs.
Browser developer applications, including Chrome DevTools, are indispensable for entrance-conclude developers. They enable you to inspect the DOM, check community requests, check out genuine-time effectiveness metrics, and debug JavaScript within the browser. Mastering the console, resources, and community tabs can change discouraging UI problems into manageable responsibilities.
For backend or method-stage developers, resources like GDB (GNU Debugger), Valgrind, or LLDB present deep control above jogging processes and memory management. Finding out these applications may have a steeper Understanding curve but pays off when debugging effectiveness issues, memory leaks, or segmentation faults.
Past your IDE or debugger, turn into snug with version Manage techniques like Git to be aware of code record, find the exact second bugs have been launched, and isolate problematic improvements.
Finally, mastering your applications means going past default settings and shortcuts — it’s about building an intimate understanding of your growth surroundings to ensure when difficulties occur, you’re not missing in the dead of night. The greater you already know your applications, the greater time you may shell out fixing the actual difficulty as opposed to fumbling by means of the process.
Reproduce the issue
Probably the most crucial — and often missed — ways in productive debugging is reproducing the challenge. Ahead of jumping into the code or making guesses, builders need to have to make a constant ecosystem or state of affairs the place the bug reliably appears. Without reproducibility, correcting a bug gets a sport of chance, often bringing about wasted time and fragile code changes.
The initial step in reproducing a difficulty is gathering just as much context as you possibly can. Ask issues like: What actions triggered The problem? Which atmosphere was it in — enhancement, staging, or creation? Are there any logs, screenshots, or error messages? The greater detail you may have, the simpler it results in being to isolate the exact conditions beneath which the bug occurs.
When you finally’ve collected plenty of info, seek to recreate the trouble in your neighborhood surroundings. This may suggest inputting the identical facts, simulating comparable person interactions, or mimicking system states. If The problem seems intermittently, think about producing automatic exams that replicate the sting cases or condition transitions included. These tests not just enable expose the issue and also reduce regressions Later on.
From time to time, the issue could be natural environment-specific — it might come about only on sure operating techniques, browsers, or underneath particular configurations. Utilizing equipment like Digital equipment, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating this sort of bugs.
Reproducing the challenge isn’t simply a move — it’s a state of mind. It needs endurance, observation, and also a methodical solution. But once you can regularly recreate the bug, you are presently halfway to repairing it. By using a reproducible circumstance, You should utilize your debugging applications extra correctly, test potential fixes safely, and communicate more Evidently with your workforce or users. It turns an summary criticism right into a concrete problem — and that’s exactly where developers prosper.
Browse and Fully grasp the Mistake Messages
Error messages are frequently the most precious clues a developer has when one thing goes Incorrect. As an alternative to observing them as aggravating interruptions, developers should master to take care of error messages as direct communications within the process. They typically let you know precisely what transpired, wherever it occurred, and occasionally even why it happened — if you know the way to interpret them.
Commence by studying the information meticulously and in comprehensive. Quite a few developers, specially when underneath time stress, look at the primary line and instantly get started generating assumptions. But deeper from the error stack or logs may perhaps lie the real root cause. Don’t just duplicate and paste error messages into search engines — read through and comprehend them to start with.
Break the mistake down into components. Can it be a syntax error, a runtime exception, or maybe a logic error? Does it issue to a particular file and line selection? What module or operate brought on it? These queries can guideline your investigation and level you towards the responsible code.
It’s also valuable to understand the terminology on the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java generally follow predictable designs, and Discovering to recognize these can substantially increase your debugging procedure.
Some glitches are imprecise or generic, and in Individuals conditions, it’s essential to examine the context where the mistake occurred. Examine linked log entries, enter values, and recent alterations during the codebase.
Don’t overlook compiler or linter warnings both. These typically precede larger sized issues and provide hints about prospective bugs.
In the long run, mistake messages are usually not your enemies—they’re your guides. Studying to interpret them appropriately turns chaos into clarity, supporting you pinpoint difficulties faster, reduce debugging time, and become a much more productive and assured developer.
Use Logging Correctly
Logging is Among the most highly effective applications inside of a developer’s debugging toolkit. When used successfully, it provides genuine-time insights into how an application behaves, helping you comprehend what’s happening under the hood without needing to pause execution or step through the code line by line.
A good logging strategy starts off with recognizing what to log and at what amount. Prevalent logging degrees include things like DEBUG, Details, WARN, ERROR, and FATAL. Use DEBUG for in-depth diagnostic information and facts all through progress, Data for basic occasions (like effective start-ups), Alert for likely concerns that don’t break the applying, Mistake for real issues, and Lethal if the program can’t carry on.
Avoid flooding your logs with abnormal or irrelevant info. An excessive amount of logging can obscure important messages and decelerate your program. Focus on vital functions, state improvements, input/output values, and critical determination points in the code.
Structure your log messages clearly and continually. Consist of context, which include timestamps, request IDs, and performance names, so it’s simpler to trace issues in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
All through debugging, logs Allow you to keep track of how variables evolve, what problems are achieved, and what branches of logic are executed—all with no halting This system. They’re Specifically important in creation environments where by stepping by means of code isn’t probable.
Furthermore, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that assistance log rotation, filtering, and integration with checking dashboards.
Finally, sensible logging is about harmony and clarity. With a properly-assumed-out logging method, you may lessen the time it will take to identify challenges, acquire deeper visibility into your apps, and Increase the General maintainability and dependability of your respective code.
Imagine Like a Detective
Debugging is not only a complex endeavor—it's a type of investigation. To properly establish and fix bugs, developers need to technique the procedure similar to a detective resolving a secret. This state of mind aids break down intricate difficulties into workable pieces and follow clues logically to uncover the root trigger.
Commence by collecting evidence. Consider the indicators of the situation: mistake messages, incorrect output, or effectiveness challenges. Identical to a detective surveys against the law scene, collect just as much applicable information and facts as you can without leaping to conclusions. Use logs, exam conditions, and person stories to piece jointly a transparent image of what’s taking place.
Subsequent, form hypotheses. Ask yourself: What could be causing this actions? Have any improvements not long ago been manufactured for the codebase? Has this problem occurred right before underneath related situation? The purpose is always to narrow down alternatives and establish likely culprits.
Then, check your theories systematically. Try to recreate the condition in a very controlled environment. When you suspect a particular function or ingredient, isolate it and confirm if The difficulty persists. Just like a detective conducting interviews, inquire your code thoughts and Allow the results direct you closer to the reality.
Spend shut focus to small facts. Bugs usually disguise inside the the very least anticipated places—just like a lacking semicolon, an off-by-one particular error, or possibly a race situation. Be extensive and affected person, resisting the urge to patch The difficulty with out thoroughly comprehending it. Momentary fixes might cover the real dilemma, just for it to resurface later on.
And lastly, maintain notes on That which you tried and uncovered. Equally as detectives log their investigations, documenting your debugging procedure can help save time for future troubles and assistance Other people fully grasp your reasoning.
By thinking just like a detective, builders can sharpen their analytical competencies, method troubles methodically, and come to be more effective at uncovering hidden troubles in elaborate methods.
Compose Assessments
Crafting checks is one of the most effective strategies to transform your debugging skills and General advancement effectiveness. Assessments not simply assistance capture bugs early but also serve as a safety net that gives you self-assurance when producing alterations on your codebase. A perfectly-analyzed software is much easier to debug as it means that you can pinpoint accurately where by and when a dilemma takes click here place.
Get started with device assessments, which center on particular person features or modules. These small, isolated checks can immediately expose no matter if a certain bit of logic is Functioning as anticipated. Whenever a test fails, you immediately know where to glimpse, noticeably cutting down enough time put in debugging. Unit checks are Primarily handy for catching regression bugs—troubles that reappear right after previously remaining fastened.
Following, integrate integration checks and conclusion-to-conclude tests into your workflow. These assistance be sure that a variety of elements of your software operate with each other smoothly. They’re specially beneficial for catching bugs that happen in elaborate programs with numerous factors or companies interacting. If some thing breaks, your checks can let you know which Element of the pipeline failed and less than what problems.
Writing assessments also forces you to Assume critically about your code. To check a function thoroughly, you will need to understand its inputs, predicted outputs, and edge cases. This amount of understanding In a natural way leads to higher code composition and fewer bugs.
When debugging a concern, writing a failing examination that reproduces the bug can be a strong starting point. Once the examination fails continuously, you are able to center on correcting the bug and view your take a look at go when the issue is settled. This solution ensures that the identical bug doesn’t return Down the road.
In short, creating assessments turns debugging from the frustrating guessing sport into a structured and predictable course of action—helping you catch a lot more bugs, speedier plus more reliably.
Consider Breaks
When debugging a tricky situation, it’s uncomplicated to be immersed in the problem—looking at your display for hrs, striving Option just after solution. But Probably the most underrated debugging resources is just stepping away. Using breaks aids you reset your thoughts, minimize stress, and sometimes see The problem from a new viewpoint.
When you're as well close to the code for as well lengthy, cognitive fatigue sets in. You may begin overlooking apparent problems or misreading code that you just wrote just hrs earlier. Within this state, your Mind will become a lot less successful at dilemma-fixing. A short wander, a espresso split, or perhaps switching to a different task for ten–quarter-hour can refresh your target. Numerous builders report acquiring the basis of an issue after they've taken the perfect time to disconnect, allowing their subconscious perform within the history.
Breaks also enable avert burnout, Particularly during for a longer period debugging periods. Sitting before a display, mentally trapped, is not simply unproductive but additionally draining. Stepping absent means that you can return with renewed Vitality and a clearer way of thinking. You could suddenly detect a missing semicolon, a logic flaw, or simply a misplaced variable that eluded you ahead of.
In the event you’re trapped, a great general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a five–ten minute crack. Use that time to maneuver around, extend, or do something unrelated to code. It could feel counterintuitive, Specially under restricted deadlines, but it really truly causes more quickly and more practical debugging In the end.
Briefly, taking breaks just isn't an indication of weakness—it’s a wise tactic. It gives your brain Place to breathe, increases your viewpoint, and will help you steer clear of the tunnel vision That usually blocks your development. Debugging is usually a mental puzzle, and rest is a component of resolving it.
Learn From Each and every Bug
Each individual bug you encounter is much more than simply A short lived setback—it's an opportunity to expand for a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural situation, every one can instruct you something useful in case you make the effort to replicate and analyze what went Incorrect.
Commence by asking by yourself some critical thoughts as soon as the bug is fixed: What caused it? Why did it go unnoticed? Could it happen to be caught earlier with much better methods like unit testing, code critiques, or logging? The answers frequently reveal blind places in your workflow or understanding and help you build stronger coding habits going ahead.
Documenting bugs can even be an outstanding practice. Hold a developer journal or keep a log where you Be aware down bugs you’ve encountered, how you solved them, and Anything you acquired. After some time, you’ll begin to see patterns—recurring issues or common issues—you can proactively keep away from.
In crew environments, sharing Everything you've learned from a bug with all your friends could be Particularly impressive. No matter if it’s by way of a Slack message, a brief compose-up, or A fast know-how-sharing session, aiding Other people steer clear of the identical problem boosts workforce effectiveness and cultivates a much better Finding out culture.
Extra importantly, viewing bugs as lessons shifts your mentality from stress to curiosity. Rather than dreading bugs, you’ll get started appreciating them as vital parts of your progress journey. In the end, a lot of the greatest builders usually are not those who create great code, but those that repeatedly discover from their faults.
In the end, Every single bug you fix adds a different layer for your ability established. So subsequent time you squash a bug, take a instant to reflect—you’ll arrive absent a smarter, more capable developer as a consequence of it.
Summary
Enhancing your debugging techniques takes time, apply, and endurance — though the payoff is huge. It can make you a far more efficient, confident, and capable developer. The subsequent time you might be knee-deep in a mysterious bug, bear in mind: debugging isn’t a chore — it’s a chance to be improved at what you do.